Translate “Animated Mouse Cursor Trail” into your language. Interested in development? Browse the code, check out the SVN repository, or subscribe to the development log by RSS. It’s like fairy dust around your page, wherever the cursor moves. Find var colour=“#000000”; now change the #000000 to whatever colour you want. Click here to see the colour codes. Get Free HTML codes and scripts. Use HTML javascript easily with HTML help and samples. Learn html source code, function, forms, website, design. If you want some sparkles around your mouse use this code. It makes explosions around your mouse cursor. Discover more posts about sparkle cursor. Anonymous asked: how can i make rainbow tinker bell sparkles follow my cursor? I tried to put the code but i guess that's the wrong one. Go here and follow the instructions. #sparkles cursor #sparkle cursor #cursor #. I am sooooo excited because I've finally, FINALLY, after a long long time, found the code for the sparkles that trail after the cursor.I've seen it before in a random blog, and I've tried to find it everywhere but to no avail.
--D. Thiebaut 08:41, 17 June 2012 (EDT)
This tutorial page explores various techniques for marking the path of animated objects in Processing. Many of the ideas and techniques presented here are taken from the Processing.org site, which remains one of the best resource for Processing. Don't hesitate to browse its pages for good ideas!
- 1Unlimited Trail
- 1.1Growing and shrinking circles
- 1.2Fading Trail
- 1.3Blurring the Trail
- 2Limited Trail
- 2.2A Trail of the Last n circles
- 2.3A More Efficient Trail
- 3Selective Transparency
Here we just start with the basic idea which we already played with in [Processing Skeleton Project and Simple Exercises | the previous tutorial in this section].
- Try it out!
- Let's play some with this simple example.
Growing and shrinking circles
- One way to make the circle grow and shrink as the animation goes on is to control its radii with a sine function whose angle is simply the number of frames since the beginning of the animation.
- The trick here relies on knowing that Processing counts the number of frames it displays and keeps the count in a variable named frameCount. The counter starts with 0, and every time draw() is called by Processing, frameCount is incremented by 1.
- Modify the program above, and add a new variable inside the draw() function:
- Because the sin() function oscillates between -1 and 1 as the angle increases, radius will oscillate between 50 - 30, and 50 + 30, or 20 and 80.
- Change the call to ellipse() and replace the constant 80 by the variable radius.
- Try your new code!
Exercise #1 |
- Make the color change with the growing and shrinking of the circle. You may find the information in the processing page on color very useful!
- Keep one of the radius values constant, and the other one equal to the variable radius.
- Use two different variables, radius1 and radius2 which are initialized the same way radius is, but use the sin() function for one, and the cos() function for the other one. Use radius1 and radius2 as the two radii value in the ellipse() function...
- Hints & Solutions
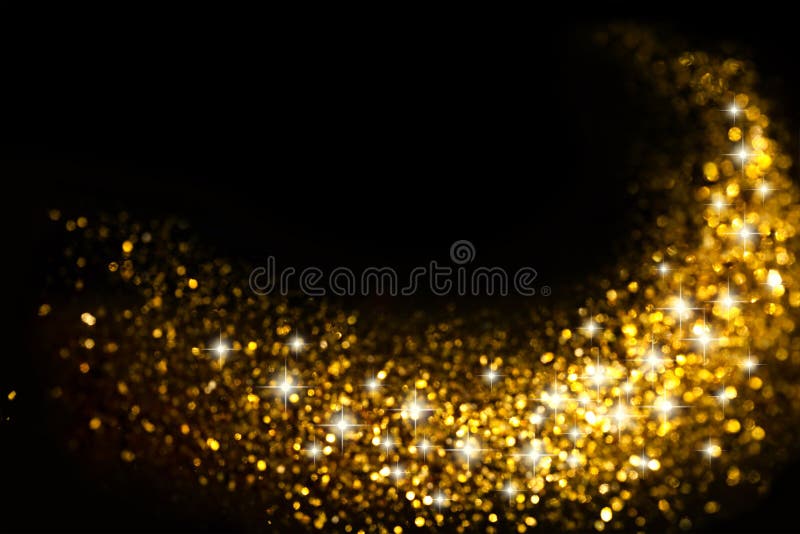
Fading Trail
One way to make the trail disappear is to make whatever is drawn in the applet become more and more transparent as it ages. In this case, objects that have been drawn in the current frame are fully opaque, while objects that have been drawn in earlier frames become more and more transparent, to the point where they disappear.
This is accomplished by painting a transparent rectangle over the whole applet before we draw new objects in the window. The objects already on the applet get more transparent as the process is repeated. All we need to do is add these three lines at the beginning of draw():
The magic number here is 50 which is the amount of transparency we give the whole applet before we draw a new circle.
By the way, if you are surprised by the expression 0xee' or 0xff startles you, don't be! It's just a different system for representing integer numbers. This format is call hexadecimal. The 0x part means that what follows are digits or letters that represent hexadecimal numbers. 0x00 is 0. 0x01 is 1. 0x09 is 9. 0x0a is 10 decimal. 0x0b is 11, 0x0c is 12, 0x0d 13, 0x0e 14, 0x0f 15, 0x10 16, ... 0xff is 255. In hexadecimal we can represent any integer between 0 and 255 with only two hexadecimal digits, between 0x00 and 0xff.
The new draw() function looks something like this:
- Try it!
Exercise #2 |
- Change the value of the transparency (50) and try different values to see how they affect the applet and the trail. The lowest acceptable is 0, the highest is 255.
- Try adding a transparency value to the circles, to see how they are affected. Example of a transparency of 100:
(For reference, a transparency of 255 is fully transparent, while 0 is fully opaque.)
- Change the background color to white, or black, and, again, play with different values of transparencies to better understand how the applet is affected.
Blurring the Trail
Another approach that is similar to the transparency approach (making past drawn objects more and more transparent as time progresses) is to continuously blur the past objects more and more until they merge with the background. This operation is carried out by the Processing filter() function. You can read some background information here. There is some good background material as well here. Read this first, then continue with this section!
Let's play! Here some code you should paste into a new class in your Eclipse project:
- See how the circles get blurred little by little, and that the last one drawn, the one that follows the mouse is always sharp?
- You will notice that little by little the screen gets darker and darker and will likely finish all black, except for the white circle. That is because we are constantly adding new black pixels in the border of the new circles, and if we do not move the mouse much, the black of the pixels, as it gets blurred, creates darker and darker pixels around, and this gray increases until the window becomes black. We'll see in one of the exercises how we can keep the window white.
Exercise #3 |
- Run the code above and move the mouse in big circles inside the applet. Keep a constant speed. See how much a delay there in Processing keeping up with you. As you move the mouse pointer around, Processing draws circles 1/2 an inch to an inch away from it, as it tries to keep up. See that?
- Now, change the line
- and replace 1 by 6. This number controls how aggressive the blurring operation is.
- Repeat the same experiment, moving the mouse pointer in circles. You should observe that this time the applet is not keeping up with you as well as before. This is because a BLUR with parameter 1 involves modifying fewer pixels around each pixel blurred, than a BLUR of 6.
- Play with different values of BLUR to better get a sense of the amount of blurring, and how this algorithm works.
- What if we still wanted to use a BLUR of 6 but make the applet more responsive? We can simply decide to blur every other frame!
- Try this in your code and see if the applet gets a bit more responsive.
Exercise #4 |
Custom Cursor Trails
- To keep the window white and prevent it from getting gray or black, just use the transparency trick we saw earlier, and add these 3 lines at the beginning of draw():
- Play with the transparency of 100. Make it larger, smaller. See what you like best as a result. Play as well with the BLUR parameter and adjust these two numbers until you get something you like.
Exercise #5 |
- Replace
- by
- and see what happens...
- DILATE is another algorithm that the filter() function can apply to the whole applet. Just another option when creating your animation and special effects!
In the limited trail case, we only draw a finite number of circles in the trail. Say 10. So, when we draw a new circle, we also draw the last 9 circles we drew last, and none of the others. So we need a data structure that will hold circles in a First In, First Out way.Processing supports ArrayList (as does Java). So we need to figure out what we need to remember for each circles. In our case, it's the x and y of the center of the ellipse, as well as its radii. The best way to do this is to create a circle class that will hold these values.
First, an Applet with A Circle Class
Study the code below and see how the new class encapsulates the circle information.
- Try out the code, and play with it!
A Trail of the Last n circles
All we need to do now is to remember the last n (say 10) circles drawn, and every call to draw we erase the applet, and draw these n circles. To remember the circles, we store them in a queue (FIFO) implemented for right now with an ArrayList.
Paste the code below in a class called Main. Study the code. See the new import statements that introduce the ArrayList and the Iterator in the applet.
- Run the code and see how we now have a trail of 10 circles.
Exercise #6 |
- Change the number of circles kept in the trail. Try large numbers, such as 100, or 500.
- Modify the program so that a transparency value is added to the color of each circle (fill and stroke), and make the transparency of the most recent (last) circle fully opaque (0), and the transparency of the oldest one (at Index 0), 255. You may find that looping through the circles in reverse order makes this simpler:
Exercise #7 |
- Instead of having the leading circle follow the mouse, make it move randomly, in a manner similar to what we explored in the previous tutorial.
Exercise #8 |
- Are you ready for a bit of challenge? Try keeping track of 2 circles, each with a trail of 10 circles...
- When you're done, try keeping track of 20 circles, each with a trail of 10 circles...
A More Efficient Trail
The previous example is fine for short trail, but ArrayList are not efficient data structures when we remove their 0th element: they shift all the remaining elements by one, which is an O(N) operation. If the trail is long, say 10,000 element, then removing the 0th one every call to the draw() function will take 10,000 steps.
The queue, which is an interface to the LinkedList collection in Java is a better suited on which to draw pixels, but to make this surface not subject to the graphics commands that are called inside the draw() function. And at the end of draw() we 'overlap' the buffer onto the applet.
An example will illustrate how this might work. Copy/paste the code below
- Play with the code. Notice how it the circles disappear while the rectangles don't. Neat, no? :-)
Exercise #10 |
Mouse Cursor Trails
- You will notice that the circles appear below, or underneath the rectangles. Change that so that they appear above the rectangles.
- Make the rectangles move randomly, rather than at a constant offset from the circles.
- Switch the behaviors of the rectangles and the circles. The circles should not disappear while the rectangles should... :-)
- Use the filter( BLUR ) method for the circles, and the filter( DILATE) method for the rectangles, simultaneously... :-) :-)
Glitter Trail Cursor Codes
Glitter Cursors For Windows 10
Voila!
Free Glitter Cursors
- How to Edit Your Tumblr [NEW] | Theme, Cursor, Scroll Bar, Add Music,Tagged Pages
Some Sites For Themes: http://baethms.tumblr.com/mintos http://shythemes.tumblr.com/ http://olleotathemes.tumblr.com/ https://leenthemes.com/tumblr-themes/ ...
Watch Now - [Tutorial] Cursor, Sparkle Trail and Music Player for Tumblr
In this tutorial, I showed you how to get cursor, sparkle trail and music player for you tumblr blog. Here are the links : Cursor links: ...
Watch Now - tumblr's pain in the ass 'uh oh! we couldn't save your theme.' & how to fix it
edit: IF IT DOESN'T WORK, MAKE SURE YOU FOLLOW THE STEPS AGAIN AND REPLACE ALL INSTANCES OF HTTP*S* _before_ REPLACING ALL ...
Watch Now - tumblr cursor codes - Edit your tumblr cursor
Get code - http://freetexthost.com/5ic34u0f4y Simple walk through method to add a rather good curser to your tumblr page.
Watch Now - [Tutorial] How To Add Cursors & Falling Glitter On Tumblr
UPDATE********** For those who are confused because of the new look for customization, take look at this new video ...
Watch Now - How to change your Tumblr cursor
go to http://www.dolliecrave.com/tumblr-cursors.php?page=1 (or you can literally type in anything on google like if you want a Justin Bieber one just type in on ...
Watch Now - How to make your own cursor for Tumblr.
You need to have: Photoshop: http://hesstillkidrauhl.tumblr.com/post/42628314186/how-to-download-photoshop-cs5-for-free MESSAGE ME OR COMMENT IF ...
Watch Now - Tumblr Cursor Tutorial
requested by some people on tumblr. follow me: http://jaimesexiado.tumblr.com/ i take cursor requests! pastebin link: http://pastebin.com/jquWbaLr.
Watch Now - How To Change Your Tumblr Theme
Like, comment, subscribe and request more tutorials! -Follow me on tumblr! : Blacksimplicityy -Detailed Instructions- -Log into your tumblr account - on a ...
Watch Now - ✰ The Ultimate Tumblr Tutorial Guide ✰
Summary: This is a massive tutorial I made on how to use Tumblr as well as how to completely edit your blog. This tutorial features a variety of different codes ...
Watch Now - How to Post Source Code on Tumblr : Web Coding Made Easy
Subscribe Now: http://www.youtube.com/subscription_center?add_user=ehowtech Watch More: http://www.youtube.com/ehowtech Posting source code on ...
Watch Now - How to change your cursor on tumblr. EASY WAY [Tumblr tutorial]
Oh well Youtube won't let me paste that code. so go here to get it. http://sarahmjfan.tumblr.com/post/32416714834/the-code-you-will-need My Tumblr: ...
Watch Now - Tumblr Tutorial: Customize your cursor
READ FIRST. Sometimes the theme doesn't support a cursor change. This means that you can't customize your cursor with THIS MEATHOD on certain themes.
Watch Now - ❤️ How to customize your Tumblr | Cursors, Music players, etc.
Hey guys! Thanks for watching! open me for details ❤ ❤ ❤ ❤ ❤ ❤ ❤ ❤ ❤ Anywhos, what's up? I know this is a quick video, i just wanted something up ...
Watch Now - how to change the cursor on tumblr
I Do cursor requests :))) hope this helps IF you dont understand or have any questions Follow these accounts and ask Follow my tumblr and ill follow you back ...
Watch Now - Tumblr Tutorial-Custom Cursor
Hi There Today ill be showing you how to play customize your cursor :) 1) Go to www.totallylayouts.com site and find and click 'Tumblr Cursors' link 2) Once you ...
Watch Now - Speed Coding - Tumblr Sidebar
Watch me code a sidebar for a tumblr theme I'm working on. Its like speed painting only instead of painting, I'm coding, and instead of a finished product at the ...
Watch Now - How to change your tumblr theme and Cursor
how to change your tumblr theme and cursor/mouse.
Watch Now - Create your own Tumblr Customized Cursors
I'm very sorry for my voice, I have colds. lol here's the link for the software: http://www.rw-designer.com/cursor-maker here's the link for the codes: ...
Watch Now - Tumblr || How to make and then add a cursor on your Tumblr blog
In HD is better ;) Little new video about Tumblr and this time no music player lol In this video I show you a way to use your own cursor on your Tumblr blog, you ...
Watch Now